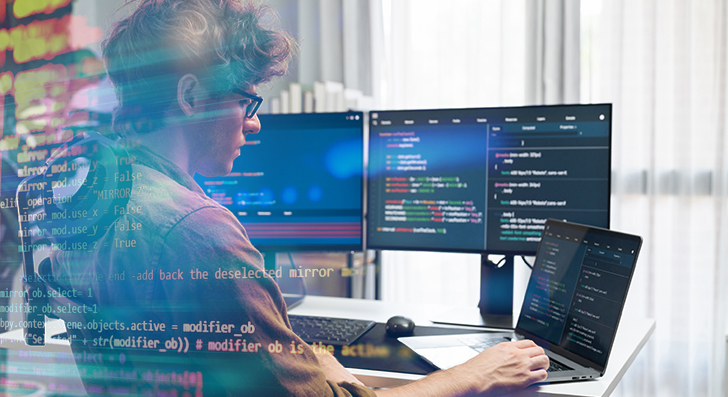
Scalability indicates your software can take care of development—more buyers, far more info, and even more traffic—without the need of breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be part of your respective strategy from the start. Numerous apps fail if they develop rapid simply because the first style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where by every little thing is tightly linked. Instead, use modular design and style or microservices. These styles crack your app into more compact, impartial pieces. Every module or provider can scale By itself without having impacting the whole program.
Also, contemplate your databases from working day 1. Will it want to manage one million users or simply a hundred? Select the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another important issue is to avoid hardcoding assumptions. Don’t create code that only functions below existing situations. Think of what would come about If the consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that help scaling, like message queues or occasion-driven techniques. These enable your application take care of a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just planning for achievement—you happen to be minimizing foreseeable future head aches. A nicely-prepared process is simpler to maintain, adapt, and grow. It’s improved to prepare early than to rebuild afterwards.
Use the best Database
Deciding on the suitable database is really a vital Component of creating scalable applications. Not all databases are constructed the same, and utilizing the Incorrect you can sluggish you down or perhaps induce failures as your application grows.
Begin by understanding your facts. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is a good healthy. They're strong with associations, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and details.
Should your details is much more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally much more easily.
Also, take into account your read and compose designs. Are you carrying out many reads with fewer writes? Use caching and browse replicas. Are you presently handling a significant write load? Explore databases which will handle large publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not require Innovative scaling capabilities now, but deciding on a databases that supports them usually means you won’t want to change later on.
Use indexing to hurry up queries. Prevent unwanted joins. Normalize or denormalize your details depending on your access patterns. And always keep track of database functionality while you increase.
In a nutshell, the best database is determined by your app’s construction, pace wants, And the way you anticipate it to increase. Just take time to choose properly—it’ll conserve a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every small delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Start by crafting clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most complicated solution if an easy a single works. Keep the functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places the place your code will take too very long to run or takes advantage of excessive memory.
Up coming, look at your databases queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really need. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you detect precisely the same details becoming asked for many times, use caching. Shop the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more successful.
Remember to check with huge datasets. Code and queries that get the job done great with one hundred information may possibly crash every time they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your application continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If every thing goes via 1 server, it'll swiftly become a bottleneck. That’s in which load balancing and caching come in. These two applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based methods from AWS and Google Cloud make this very easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same info all over again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You'll be able to provide it from your cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents near to the person.
Caching lowers databases load, improves velocity, and tends to make your application more productive.
Use caching for things which don’t modify normally. And usually ensure that your cache is updated when knowledge does change.
Briefly, load balancing and caching are easy but strong tools. With each other, they assist your application deal with far more buyers, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Resources
To create scalable apps, you would like resources that allow your app improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess foreseeable future ability. When website traffic improves, you could increase more resources with just a few clicks or automatically using auto-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply solutions like managed databases, storage, load balancing, and safety resources. You are able to focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, out of your laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, applications like Kubernetes allow you to take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual parts of your application into providers. You can update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container equipment implies you can scale rapidly, deploy easily, and Get well quickly when troubles happen. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They save time, minimize possibility, and assist you to keep centered on constructing, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when matters go Improper. Checking can help the thing is how your app is executing, place challenges early, and make better choices as your app grows. It’s a critical Element of developing scalable techniques.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and the place they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, often right before people even observe.
Monitoring can also be helpful when you make variations. When you deploy a whole new function and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of trouble until eventually it’s way too late. But with the proper instruments in place, you remain on top of things.
In a nutshell, checking will help you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Ultimate Thoughts
Scalability get more info isn’t just for significant organizations. Even compact apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel major, and Develop sensible.